Building a Salesforce API wrapper with JS part 1—project structure
There's no better way to learn Node.js and the Salesforce API than creating a clone of jsforce, the semi-official JavaScript/Node.js wrapper for the Salesforce API.
I actually already did this when I created sfdc-happy-api, which is my own wrapper that I use in HappySoup.io. Shocking...I've actually never used jsforce!
Why are we doing this
As I said, it's a great way to learn and it's fun. However, if you are creating a real fullstack Salesforce app, maybe go with jsforce as it has a ton more features and it's been tested by thousands of other apps.
I created my own wrapper for HappySoup.io because I wanted to learn how to do it.
Project structure
The focus of part 1 will be setting up the project structure for Node.js. This is actually a template that I use for all my Node projects and it's worked wonders for me.
So we start off by creating a node project using the npm init
command and going through the steps. Just give the project a name and leave the default values for the rest
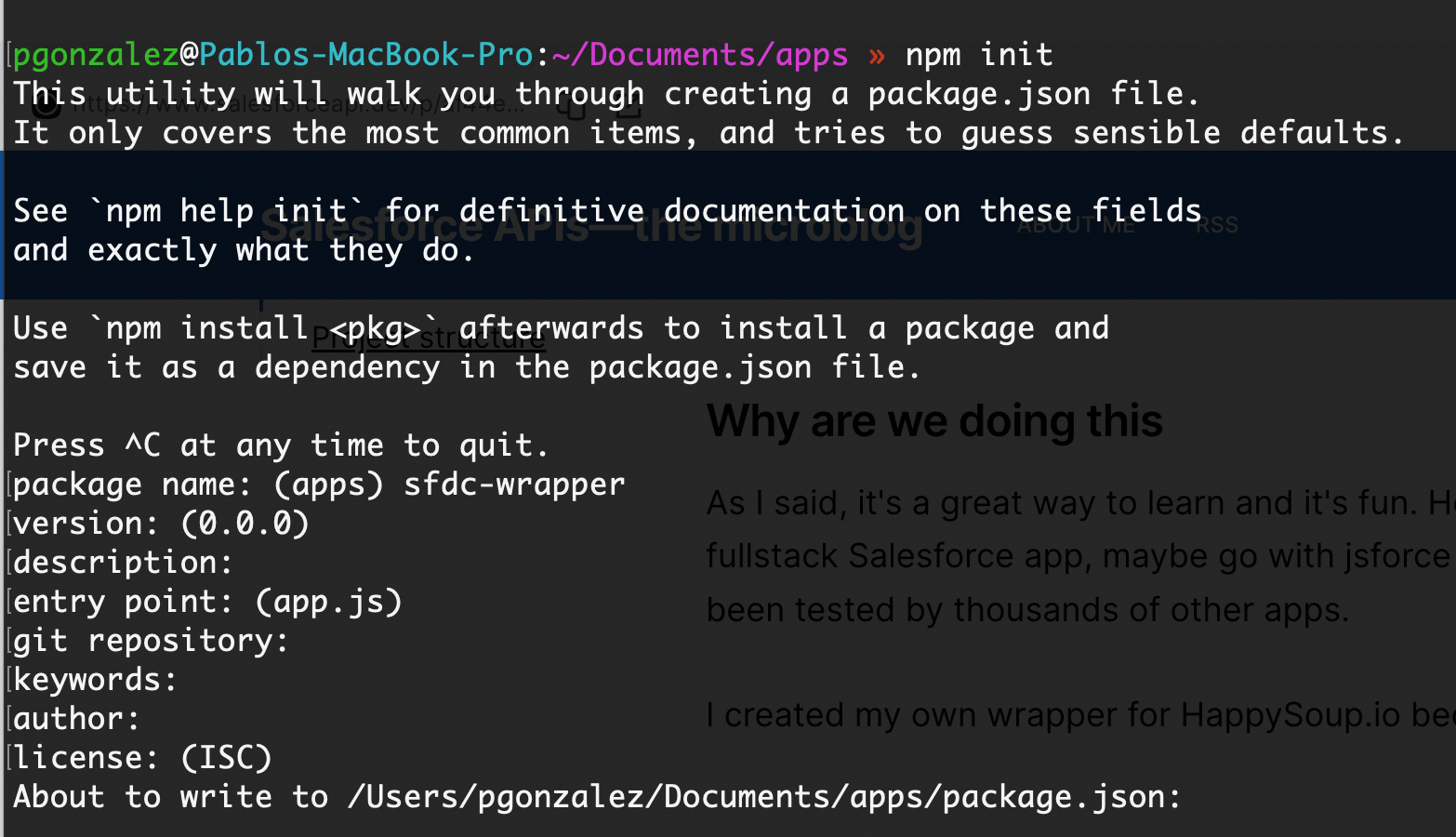
Now I'm going to open the new folder in vscode.
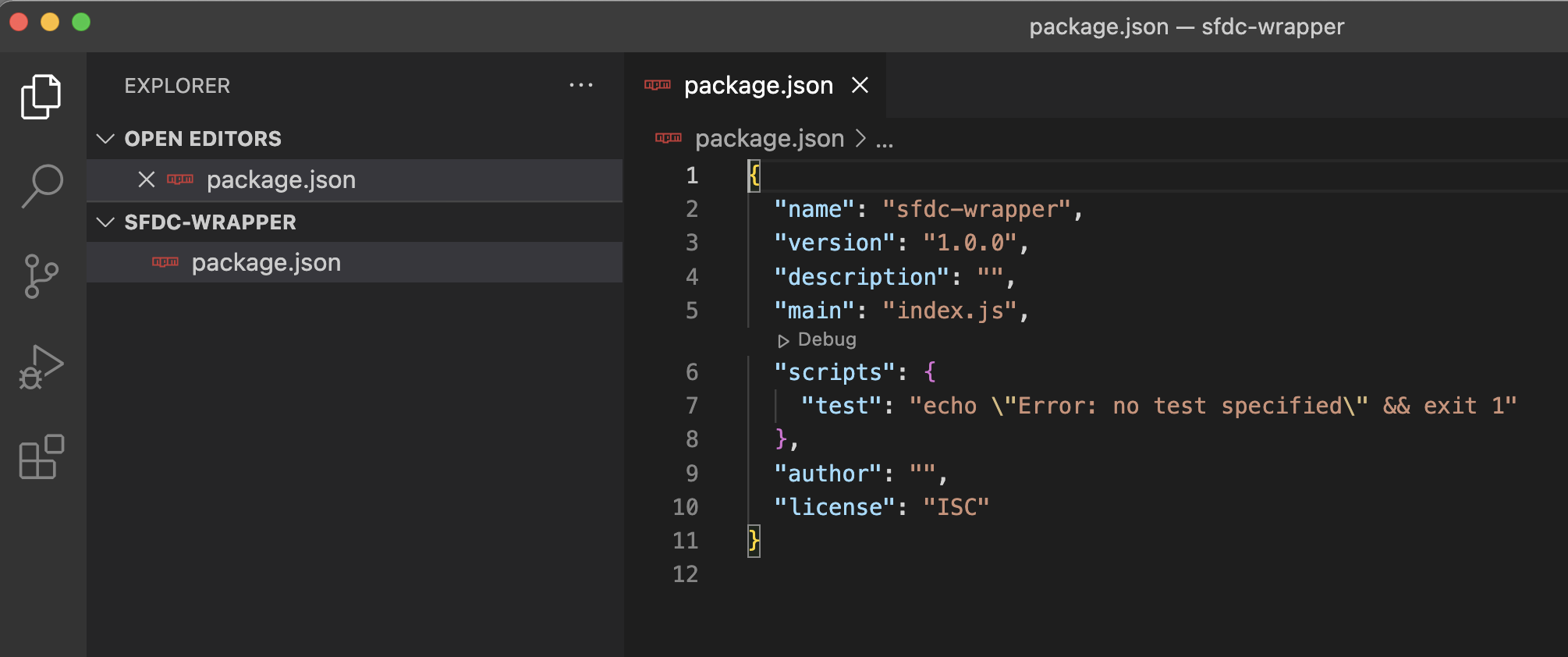
src and lib folders
Now I'm going to create 2 folders, src
and lib
using the following command
mkdir src lib
Now your project should have the following structure
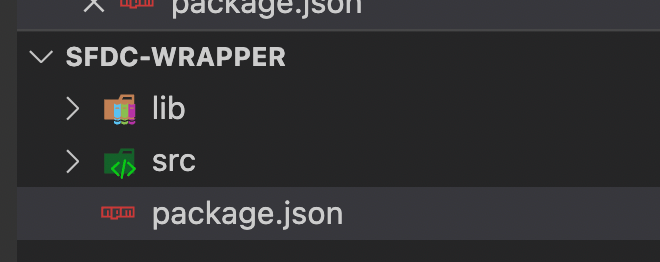
The src
folder is going to contain a index.js
file that is going to be the main entry point of our application. This is the file that gets things moving. You can think of it as your main
method in Java (if you are familiar with that).
The lib
folder is where we add other libraries or helper functions. I generally put most of my logic here and I keep index.js
as clean as possible.
So in the case of a Salesforce API wrapper, index.js
will contain very little logic. And all the API implementation, wrappers, HTTP calls, etc., will be inside multiple files in the lib
folder.
You can see this is how I've structured my wrapper
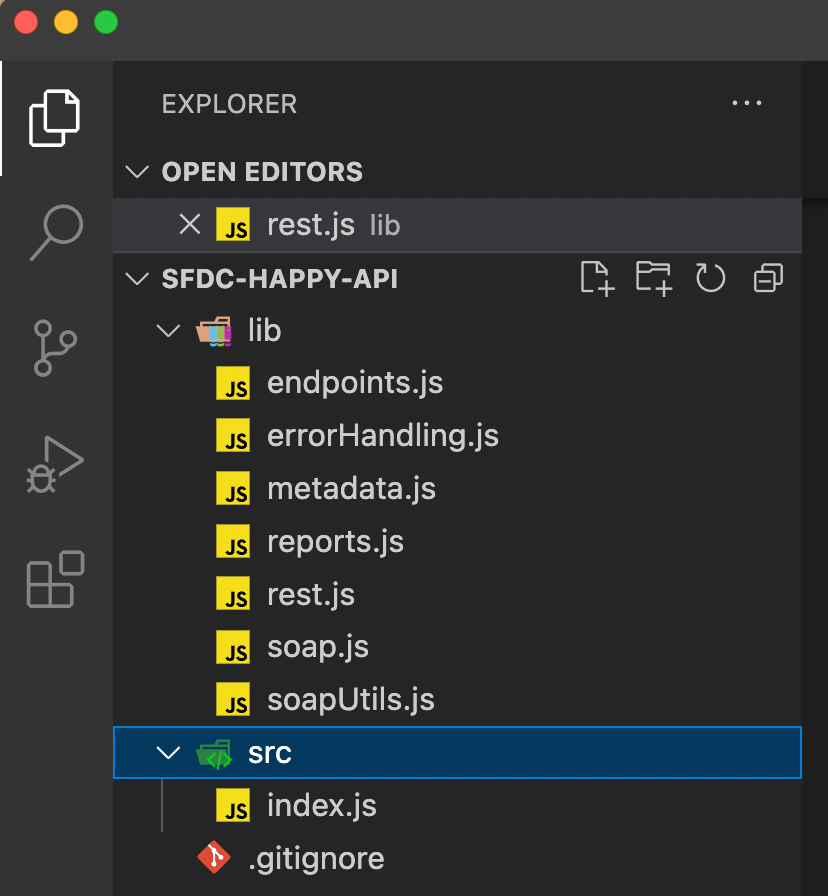
Entry point
Now, inside index.js
, I'm going to create an entry point function and export it as a module
function mainFunction(){
console.log('entry point being executed');
}
mainFunction()
module.exports = mainFunction;
Helper
Now, I'm going to create a helper.js
file under the lib
folder, and do the same
function helper(){
console.log('core logic is being executed');
}
module.exports = helper;
Putting it all together
Now, I'm going to import the helper function into my index.js
file so that I can use it here
let helper = require('../lib/helper')
function mainFunction(){
console.log('entry point being executed');
helper();
}
mainFunction();
module.exports = mainFunction;
Finally, I need to go to the package.json
file and specify which file should be executed when the program is run.
To do this, I'm going to modify the main
property to point to src/index.js
and I'm going to create a start
script that will execute the index.js
file
{
"name": "sfdc-wrapper",
"version": "1.0.0",
"description": "",
"main": "src/index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start":"node src/index.js"
},
"author": "",
"license": "ISC"
}
Now, I can use the npm start
command in the terminal, and I can see that the entry point is executed and that it calls the helper function
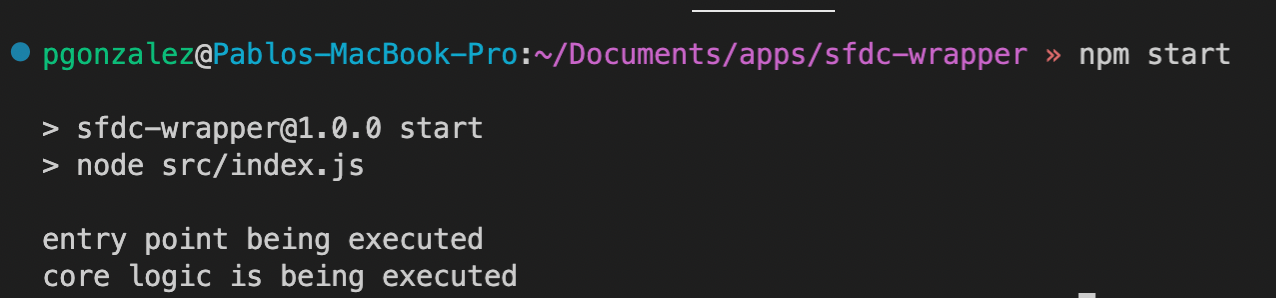
Now, this is just an example of the template I used for my wrapper. Mine as a little different, but I've found this to work great for all my NPM projects.
I've uploaded the template so that you can download it and use it yourself.
In the next post, we'll start looking at our implementation of the REST API!
Do you have any comments or feedback? Leave a comment! I read each and every one of them!